The Xcode 4.2 Review
posted on
The great Apple software release of 2011 happened a few weeks ago, bringing the likes of iOS 5 and iCloud. But we don't really care about those in this post, what we care about is Xcode 4.2. If Xcode 4.1 was the Lion release, Xcode 4.2 is the iOS 5 release (although many of the improvements apply to the Mac as well). So lets get cracking and see what's new and improved:
Storyboards
The big new feature for iOS developers is Storyboards. Apple obviously noticed that the majority of applications can be defined as screens and the transitions between them. Unfortunately it was hard to design and structure these, requiring the UI to be split across multiple NIBs and transitions to be handled in code. This ended up requiring a lot of what was effectively boilerplate code to control the flow of your app. Storyboards aims to simplify that.
The easiest way to sum up Storyboards is "all your NIBs on one canvas". It is a higher level view that essentially lets you design and view the UI and flow of your entire app in one screen (and indeed you can zoom out on a Storyboard when you want to see your entire app on one screen). I have talked a lot about the potential features that could be enabled by the improvements introduced in Xcode 4 and Storyboards is a shining example of this. There are 3 key concepts with Storyboards: Scenes, Segues and Relationships.
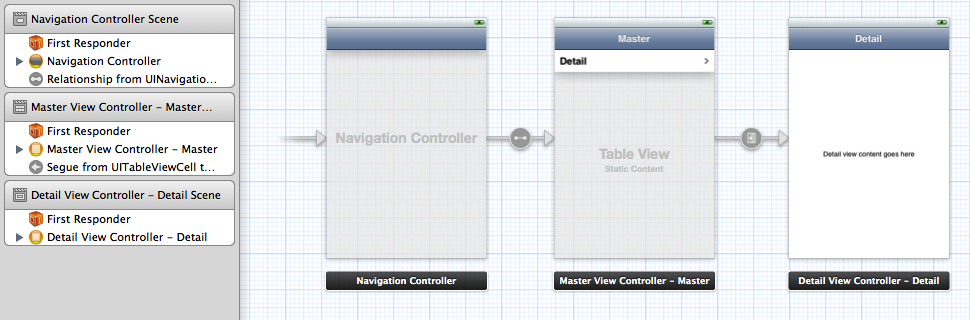
Scenes are effectively your NIBs. You create them by dragging view controllers to your Storyboard. You can design the UI in their view just like you would any NIB, and below the view you get a dock just like your NIB to hook up connections. Segues are the transitions between scenes. You just drag from a UI element in one scene to another scene and choose the type of segue to use. Built in segues include pushing onto a navigation view, showing a view modally, showing a view in a popover or just replacing the view. You can also create your own custom segues if you have your own transitions you'd like to do. Finally there are relationships. A view controller will have a relationship to content views, for example a split view has master and detail sections. Relationships simply say which view controller relates to which section.
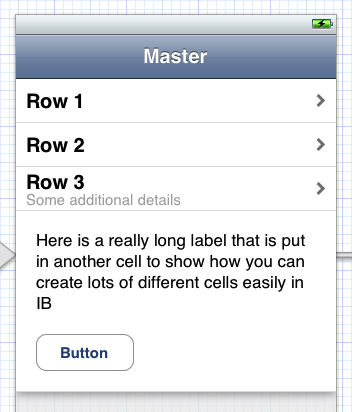
Tables
Storyboards also simplify table views. There are two types of tables you can build. Dynamic prototype tables allow you to design your different cells within the actual table, much like view based NSTableViews on the Mac. You drag your cells in, lay them out in the NIB and give them a reuse identifier. The data source code is pretty much the same, except you no longer have to manually create cells in code, you always get one back from the table.
The second type of table is a static table. Many applications use tables for static lists, but even though they never change you have to write all the code for the datasource. Static tables lets you create these in a NIB. The datasource is handled for you, so you can focus on designing and laying out the table rather than feeding up basic content.
All in all, Storyboards is probably the biggest new feature in terms of code you no longer have to write since iOS was released. I suspect it will quickly become the default way to build iOS apps, though how well it handles more complex apps remains to be seen.
NIB Editing
Storyboards aren't the only improvement for UI editing, the general NIB editing features have seen a lot of nice improvements. There is support for the new appearance APIs in iOS 5, letting you set tint colours and images for most controls. You can now also configure gesture recognisers on views from within the NIB, again saving you lots of code over previous versions.
Autolayout sees some improvements. The constraints section no longer closes every time you edit a constraint and there is now a visual distinction in the list view between the constraints you manually set and those generated by IB. Unfortunately you still cannot set negative constraint constants, despite it being perfectly valid in the API.
Finally the assistant editor sees a nice improvement with connection points appearing in the gutter next to code you can connect to. This means you can finally drag from code to objects.
LLVM 3.0
One of the most significant changes in Xcode 4.2 is in the compiler department. It seems a long time ago that we first saw the Apple LLVM Compiler 1.0 in Xcode 3.2. It promised faster compile times, faster binaries and better error messages. This compiler has moved on to become the cornerstone of many of the technologies Apple uses and builds, such as Open GL, Open CL and Xcode 4. Many of the changes seen in Xcode over the past 12-18 months have been made possible due to adopting Clang and LLVM.
Xcode 4.2 brings us version 3 of the LLVM compiler (it's worth noting that Apple's version numbers are more for Clang than for the LLVM backend, which is currently at version 2.9). For Cocoa developers the biggest change here is the introduction of Automatic Reference Counting. I won't go into ARC into too much detail here, but essentially it gives you the benefits of a garbage collector, without all of the downsides. I will say that from my experience it requires more work to use than the Objective-C GC, but it's a nice improvement to have. ARC is enabled by default in all new Xcode projects, and is easy enough to turn on via a compiler flag. There is also a refactoring option to upgrade your existing code as you desire.
Other improvements include support for the C++ '11 standard. It isn't a full implementation yet, but it is a start. And for those who are interested in code coverage, LLVM 3 also adds in support for generating gcov data files. The static analyser has seen improvements as well, with support for C++ and Obj-C++ files, as well as the ability to customise what checks are performed in the build settings of a project.
Finally, we say goodbye to a compiler. Historically GCC has been the backbone of Apple's developer tools, but now the transition away is complete. Xcode 4.2 ships with just two compilers: LLVM-Clang and LLVM-GCC. If you want GCC itself you'll have to build it from source or download from elsewhere (which is probably a better option anyway as Apple's GCC fork is rather behind). But for Cocoa development you'll have to at least adopt LLVM-GCC, but preferably LLVM-Clang as I suspect LLVM-GCC might only be an option for another few years, much like classic or rosetta, in order to aid the transition.
Debugging
The new hotness in debugging is aimed at those working with OpenGL ES. I won't pretend to know what I'm talking about as I have only used OpenGL at university (something I keep meaning to correct when I get the time), so most of this will be what I have understood from Apple's presentations.
The OpenGL ES Debugger allows you to capture a frame from an app running on a device. You do this by clicking a button in the debug bar in Xcode. It will then bring up this frame in a debugger and allow you to see everything used to build that frame. You can see all the draw calls (and optionally have them grouped by using some OpenGL ES extensions Apple have provided) and step through them one by one to see how your frame is built. Xcode will even highlight which part of the frame is currently being worked on in the main display. On top of this you can use the assistant to inspect various resources that are relevant to the project or are bound to the frame, such as textures and shaders.
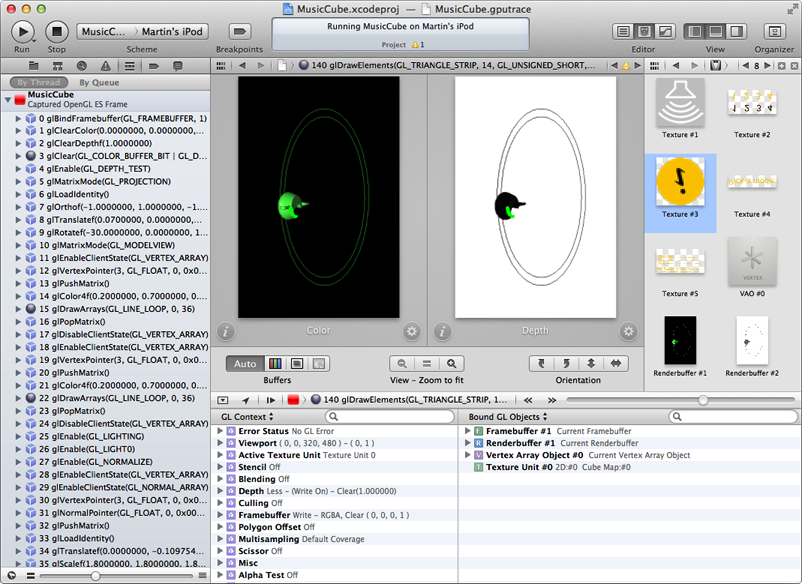
There are lots of other improvements to debugging as well. The most important of these is that LLDB actually seems stable enough to use for once. Previous versions have caused Xcode to crash pretty quickly, but I've actually been able to get some debugging done with LLDB. Another nice improvement is a menu item to continue debugging to the line the text cursor is on, which previously required the mouse to do.
For iOS development, application data can be stored in archives and loaded onto the device at runtime to aid in debugging and testing. We also finally have the ability to fake location data, allowing us to change the location to anywhere in the world, or even set up paths, which saves a lot of trips out that were required previously. There is an option to detect wireless connected devices, but I haven't actually been able to get this working, nor find any real information on it, so I'm not entirely sure whether it allows for the fabled wireless debugging or not.
Miscellaneous Improvements
These are the improvements I've noticed that don't really fit anywhere else. Firstly the preferences window has had a bit of a re-arrange. There is a new Downloads section, where you can download doc sets, but also now support for older OSs and SDKs for testing and debugging purposes. The Source Trees section has also been moved into Locations to tidy things up a bit.
There are improvements when creating new projects and files. You can specify the class prefix to use for the initial files in your app. And Apple has added the name field for creating classes and categories back, preventing all those times when you'd type your class name in the subclass field by mistake.
While iCloud is one of the big new products Apple has released, the changes to Xcode to support it are rather minimal, with a new section added to the entitlements to enter your iCloud containers.
A new feature I know many people will absolutely love is the return of sorting in the navigators. You can now sort items by type or alphabetically. There are also some improvements in code completion. Autocompletion of block parameters with void return types and arguments no longer puts in the unnecessary voids, and if you're changing an #import, the remainder of the file name will be automatically deleted when you complete, useful for the many times you end up with a stray .h
For users coming from other IDEs such as Visual Studio or Eclipse, where they're used to double clicking to open a file, Xcode 4.2 adds a preference to treat a double click as a single click, which should hopefully help make the transition easier.
Finally the organiser has seen some improvements. Macs are now shown in the devices panel, allowing them to be easily added to the developer portal. And the documentation viewer is MUCH faster. It's still slow in places but it's a dramatic improvement over 4.1.
Missed from Xcode 4.1
Sometimes after doing my reviews I'll miss some new feature that was added. There are two of these that I know about from Xcode 4.1 so I thought I would give them an honourable mention here. The first is an improvement in selecting fonts. Rather than bringing up the full font panel straight away, Xcode 4.1 introduced a popover providing a quick way to change the font, including selecting various system font styles.
Another useful change is in Core Data model files. They were previously in a binary format, which are always a joy to use with version control. If you set the tools version on your model to Xcode 4.1 it will update it to a new XML based format, making it much easier to merge any edits.
Conclusion
Xcode 4.2 is a pretty sizeable improvement. It feels noticeably more stable and faster in several key areas, and many minor niggles have been fixed. New features like Storyboards and customising control appearance should dramatically change how iOS apps are built and allow you to remove huge amounts of code. And finally the removal of GCC in favour of LLVM 3.0 signals yet another break from the past of Apple's developer tools, one that will be complete when LLDB replaces GDB.
Surprisingly I've had no radars to file for Xcode 4.2 as of yet. Those issues I've found are ones that affected previous versions and have been filed for a while. This version shows how Xcode 4 has matured. It's no longer the brand new, rather exiting albeit very buggy IDE we saw released at the start of the year. It's more stable, more complete and is starting to showcase the sorts of features that the changes made possible. This is likely the last major Xcode release, and therefore the last review, of 2011. I'm looking forward to what Apple will give us in 2012 and how it will build on the successes of this year.